Tutorials · 8 min read
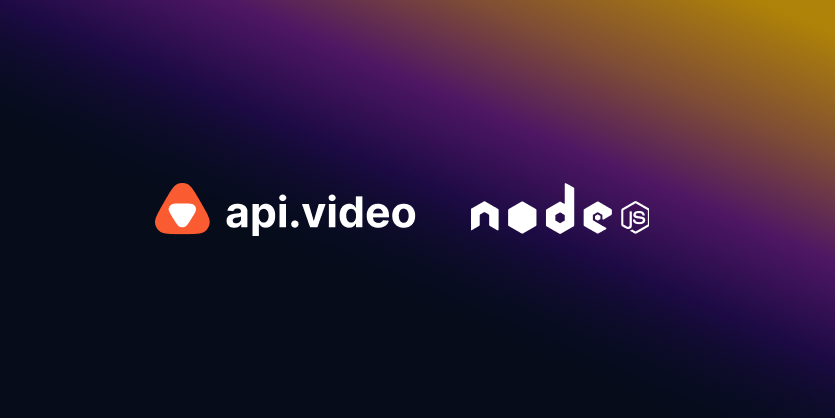
Building a video upload demo with Node.js and api.video
Using our APIs (and our SDKs) are a straightforward and easy way to add video to your service.
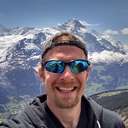
Doug Sillars
February 1, 2022
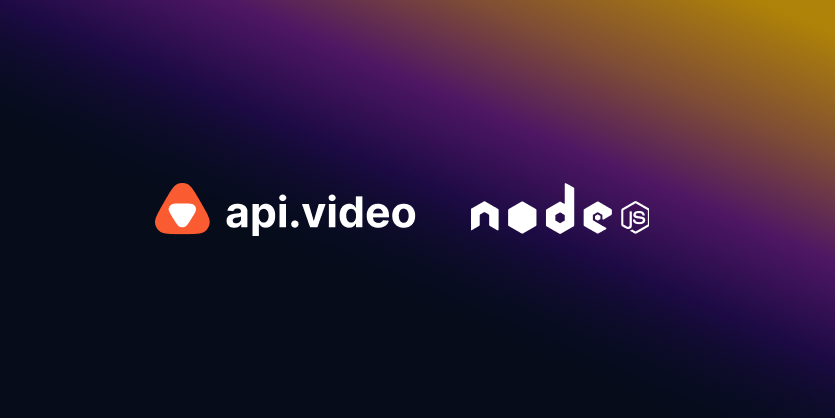
c
javascript
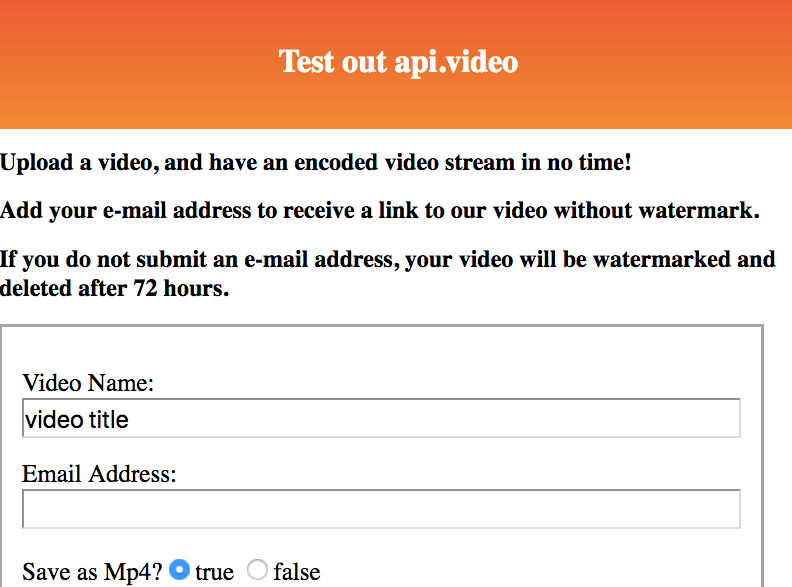
javascript
javascript
javascript
javascript
javascript
javascript
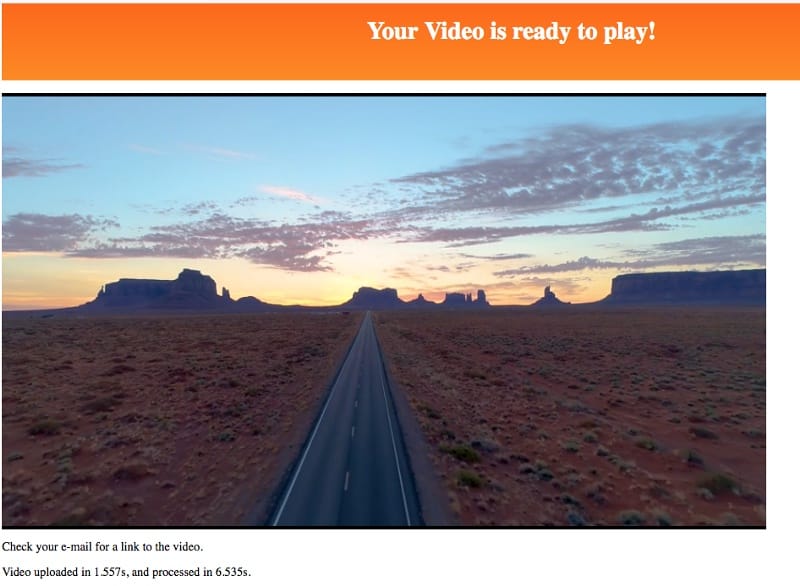
javascript
LATEST ARTICLES
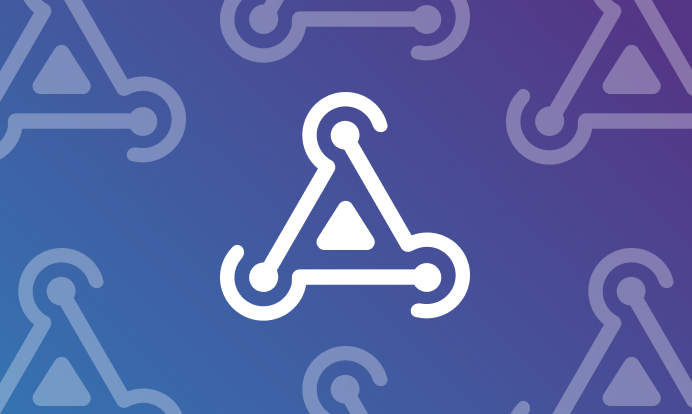
Tutorials · 8 min read
What is a webhook and how to use webhooks for real-time video management
Answer critical questions like what are webhooks, how they work, and how you can use them for video management.
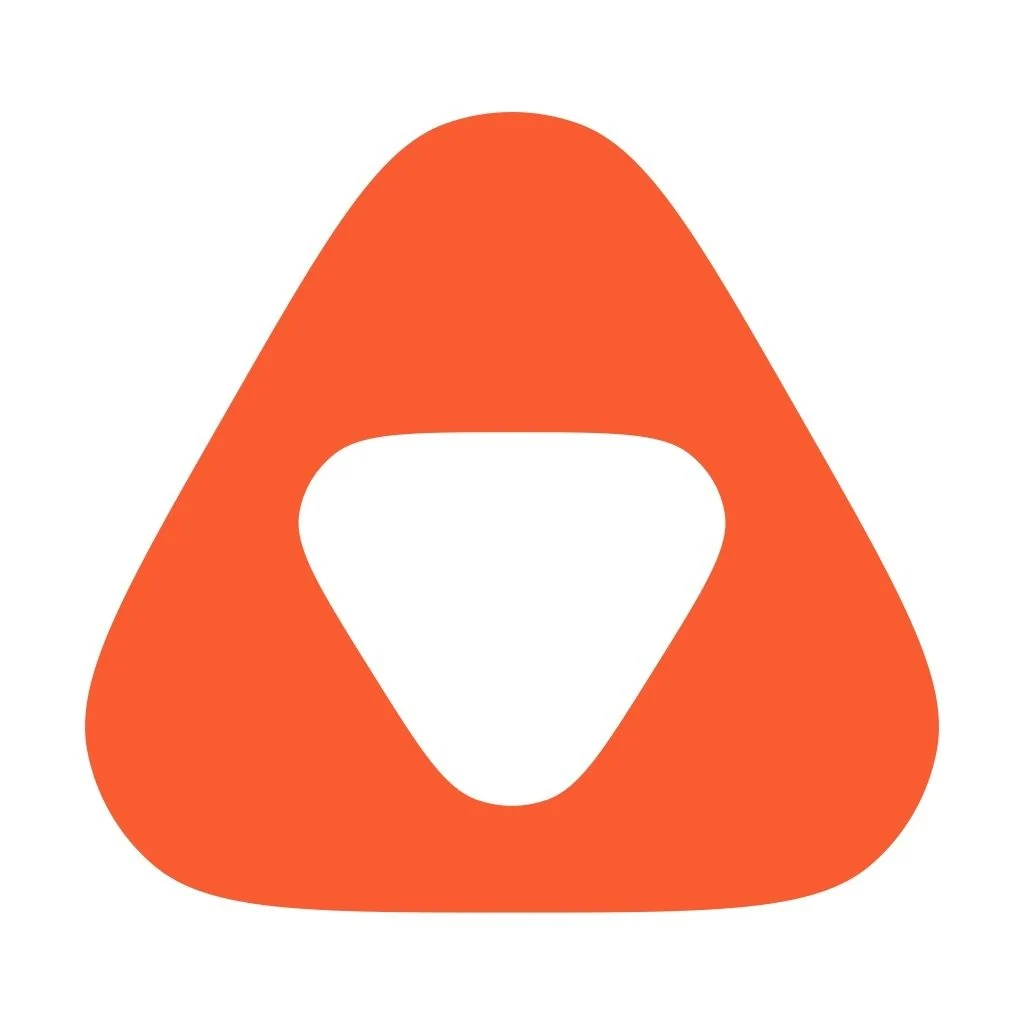
api.video · September 12, 2024
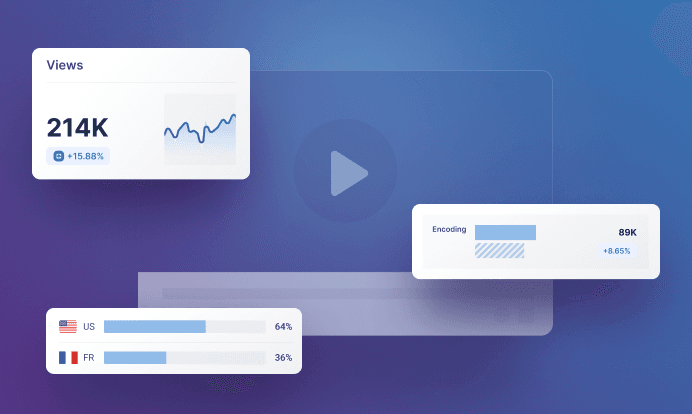
Tutorials · 6 min read
Leverage api.video’s Analytics to enhance your content strategy
Take a look at the two methods you can use to retrieve data for your videos and live streams with Analytics.
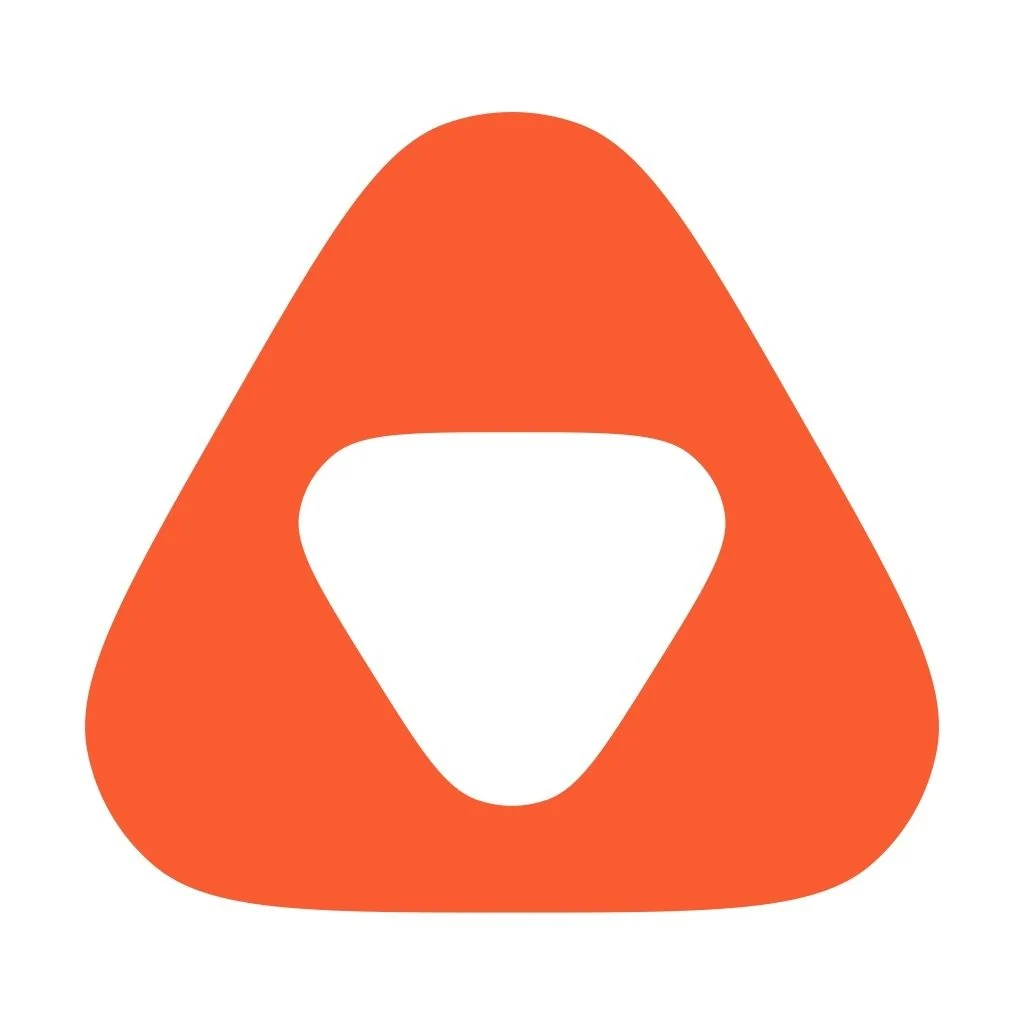
api.video · July 22, 2024
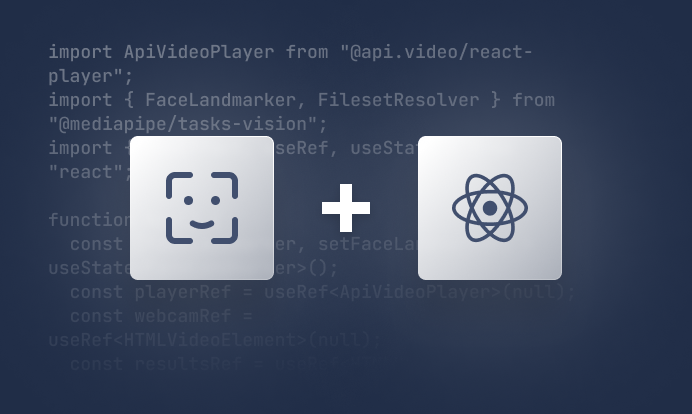
Tutorials · 4 min read
How to build AI facial expression detection into your videos with React
Learn how to add AI facial expression detection to your videos using one of Javascript's powerful libraries, React.
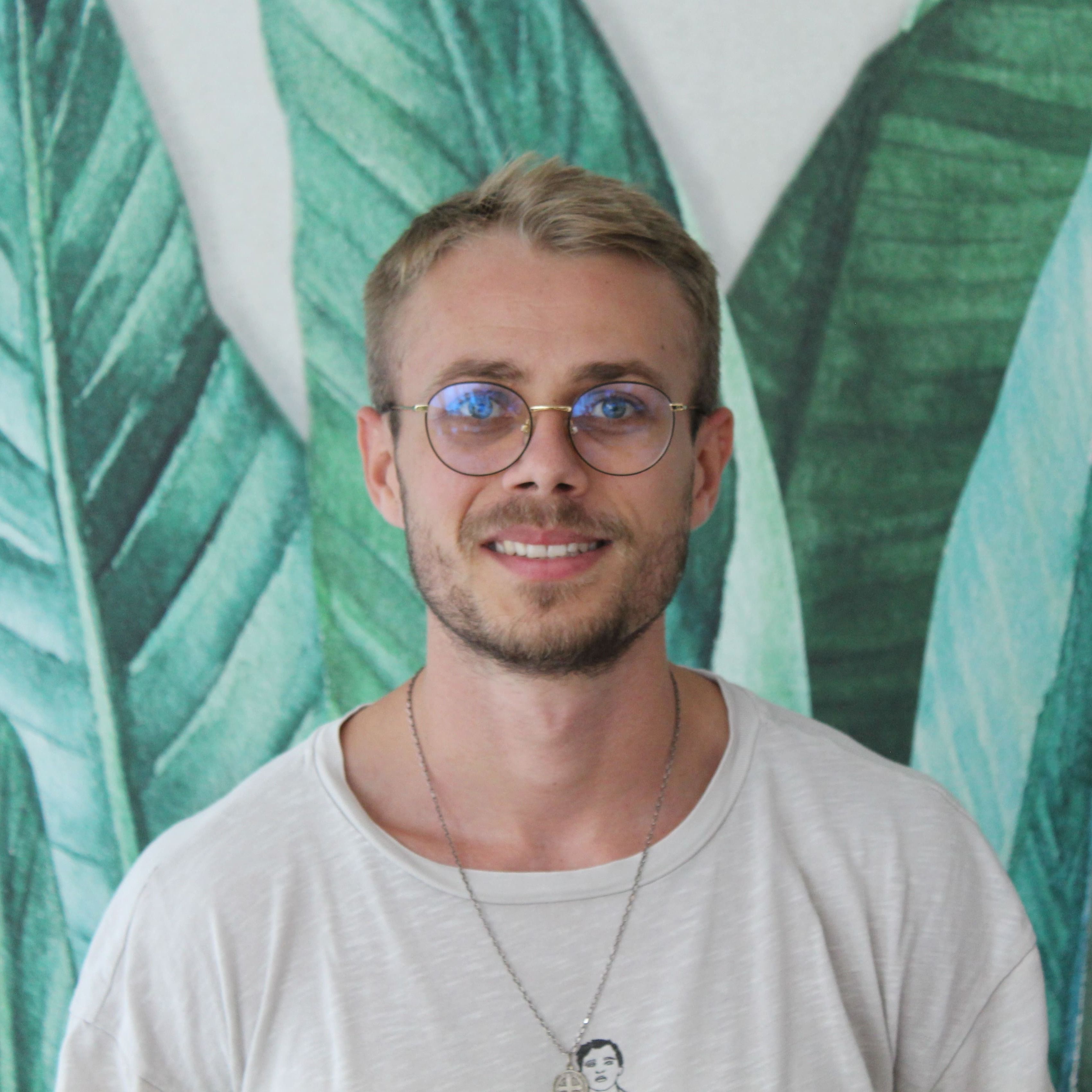
Yohann Martzolff · June 26, 2024
Try out more than 80 features for free
Access all the features for as long as you need.
No commitment or credit card required
Video API, simplified
Fully customizable API to manage everything video. From encoding to delivery, in minutes.
Video API, simplified
Fully customizable API to manage everything video. From encoding to delivery, in minutes.
Built for Speed
The fastest video encoding platform. Serve your users globally with 140+ points of presence.
Built for Speed
The fastest video encoding platform. Serve your users globally with 140+ points of presence.
Let end-users upload videos
Finally, an API that allows your end-users to upload videos and start live streams in a few clicks.
Let end-users upload videos
Finally, an API that allows your end-users to upload videos and start live streams in a few clicks.
Affordable
Volume discounts and usage-based pricing to ensure you don’t exceed your budget.
Affordable
Volume discounts and usage-based pricing to ensure you don’t exceed your budget.