Tutorials · 9 min read
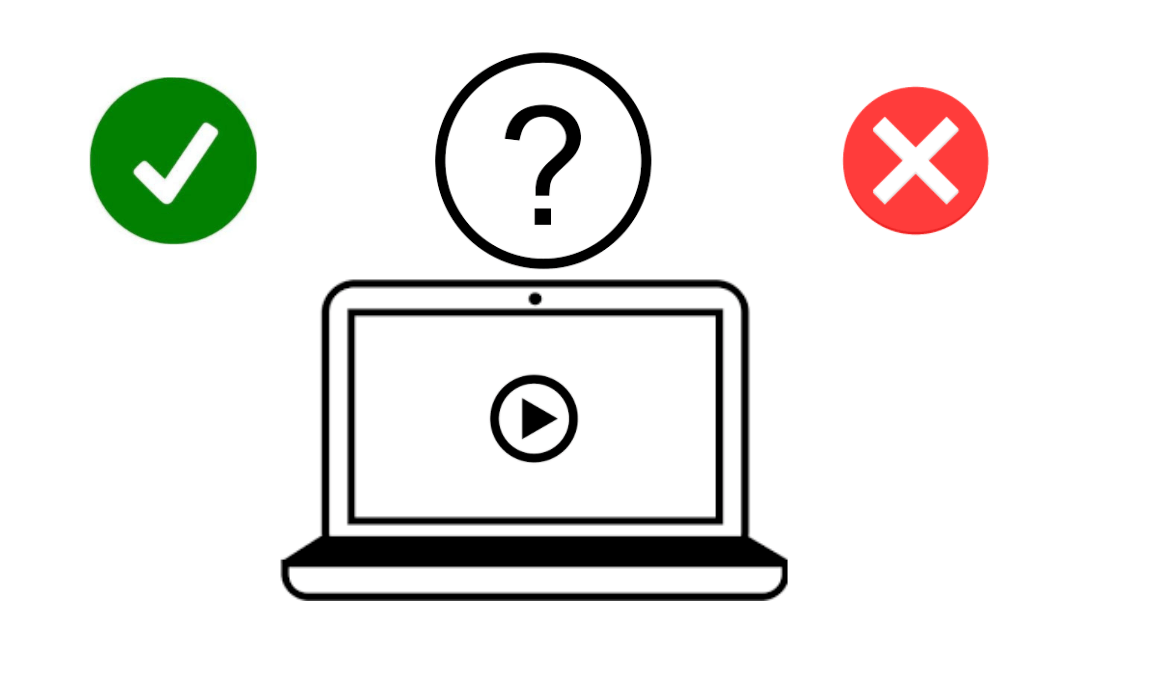
Video moderation with machine learning
Are you losing sleep thinking about the videos your customers are uploading to your site? In this post, we'll walk through a demo application that incorporates video moderation with AI, enabling you to categorise all uploaded content, and only display the content that is appropriate for your customers.
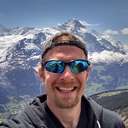
Doug Sillars
November 13, 2020
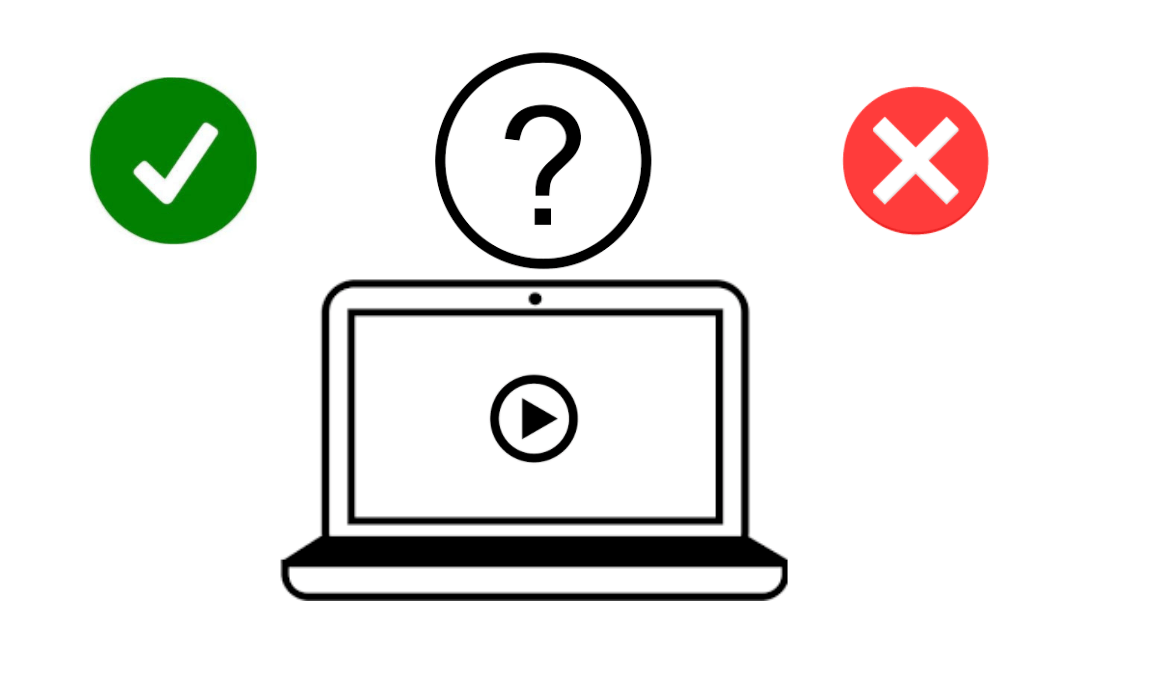
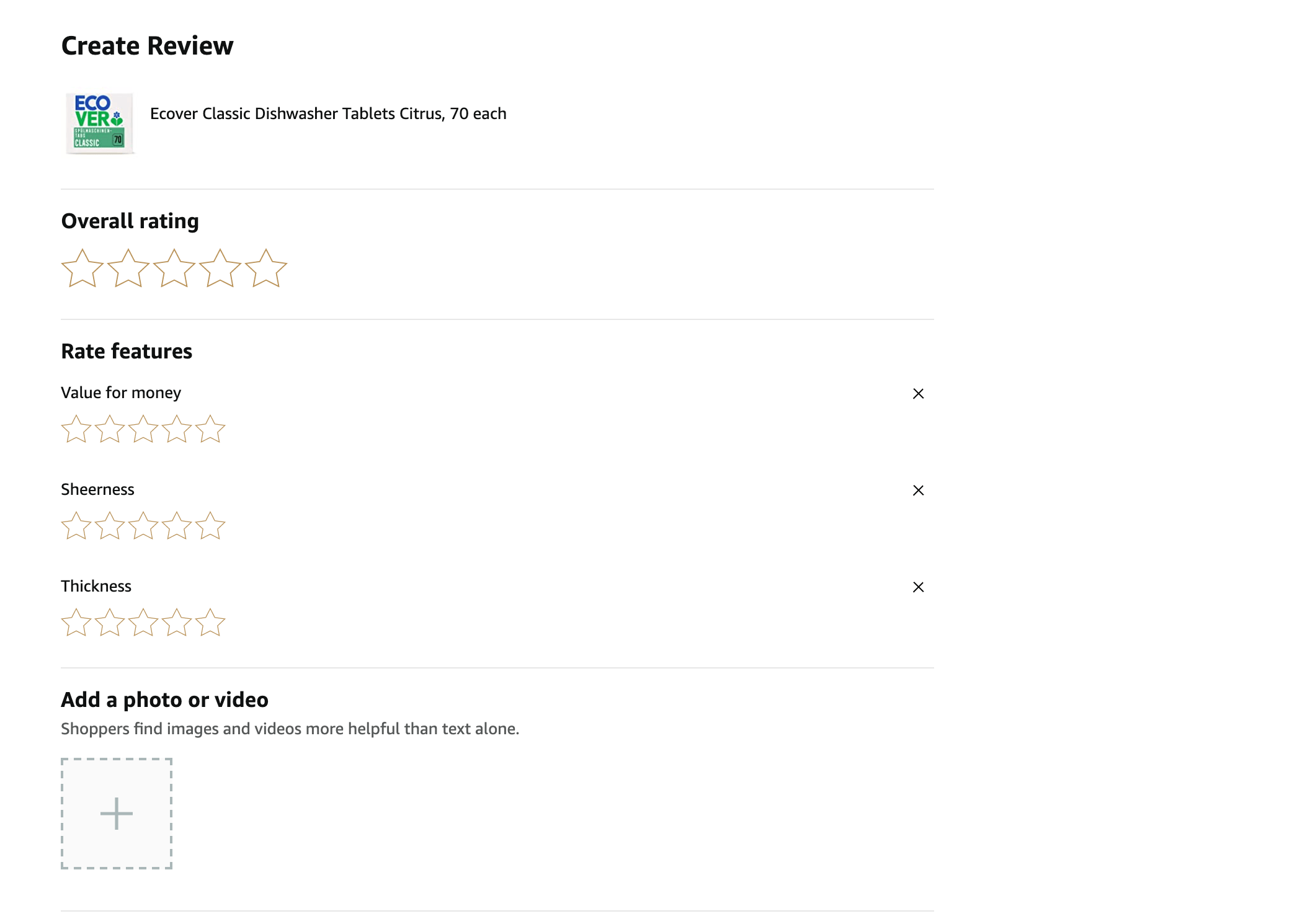
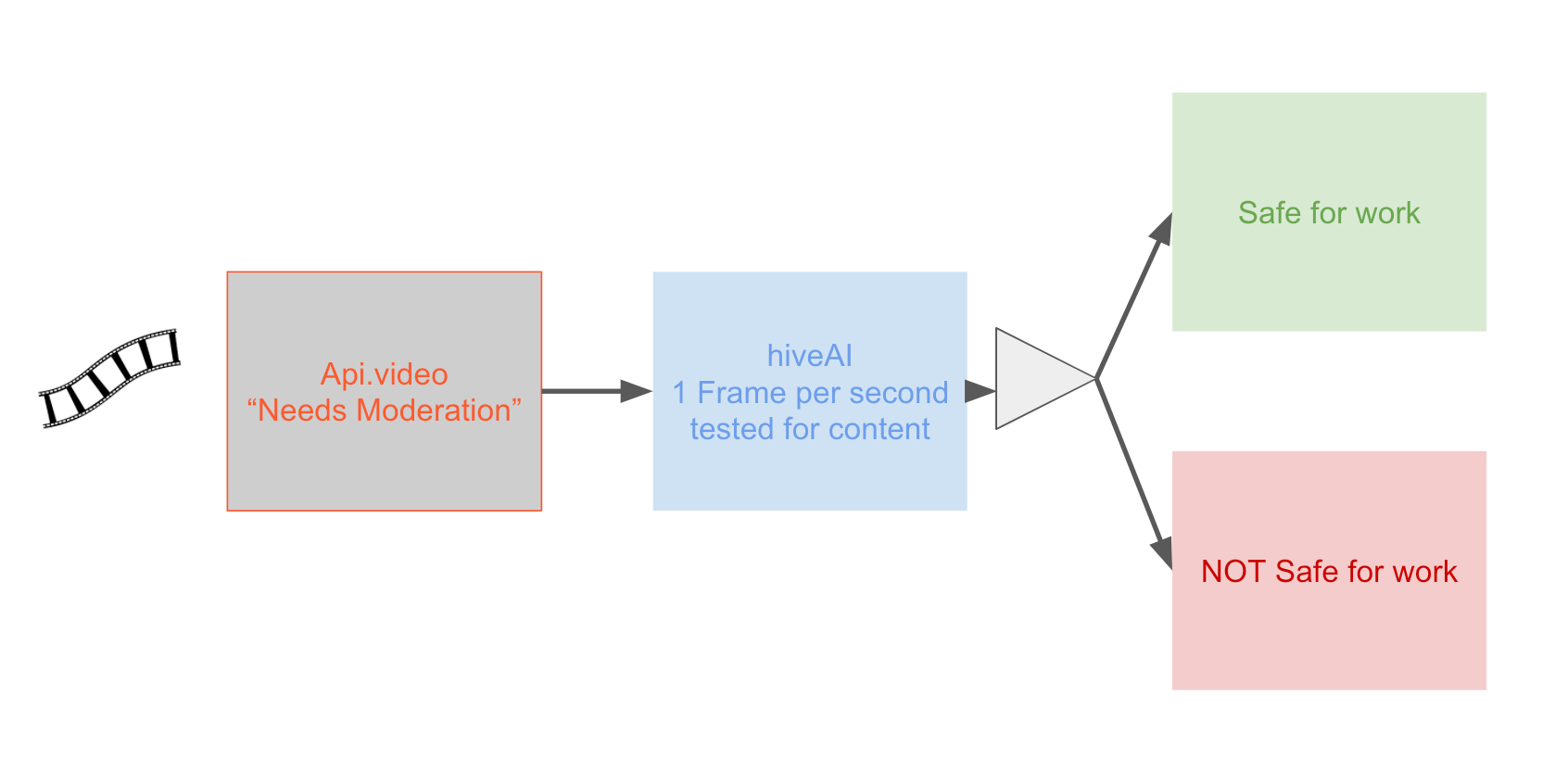
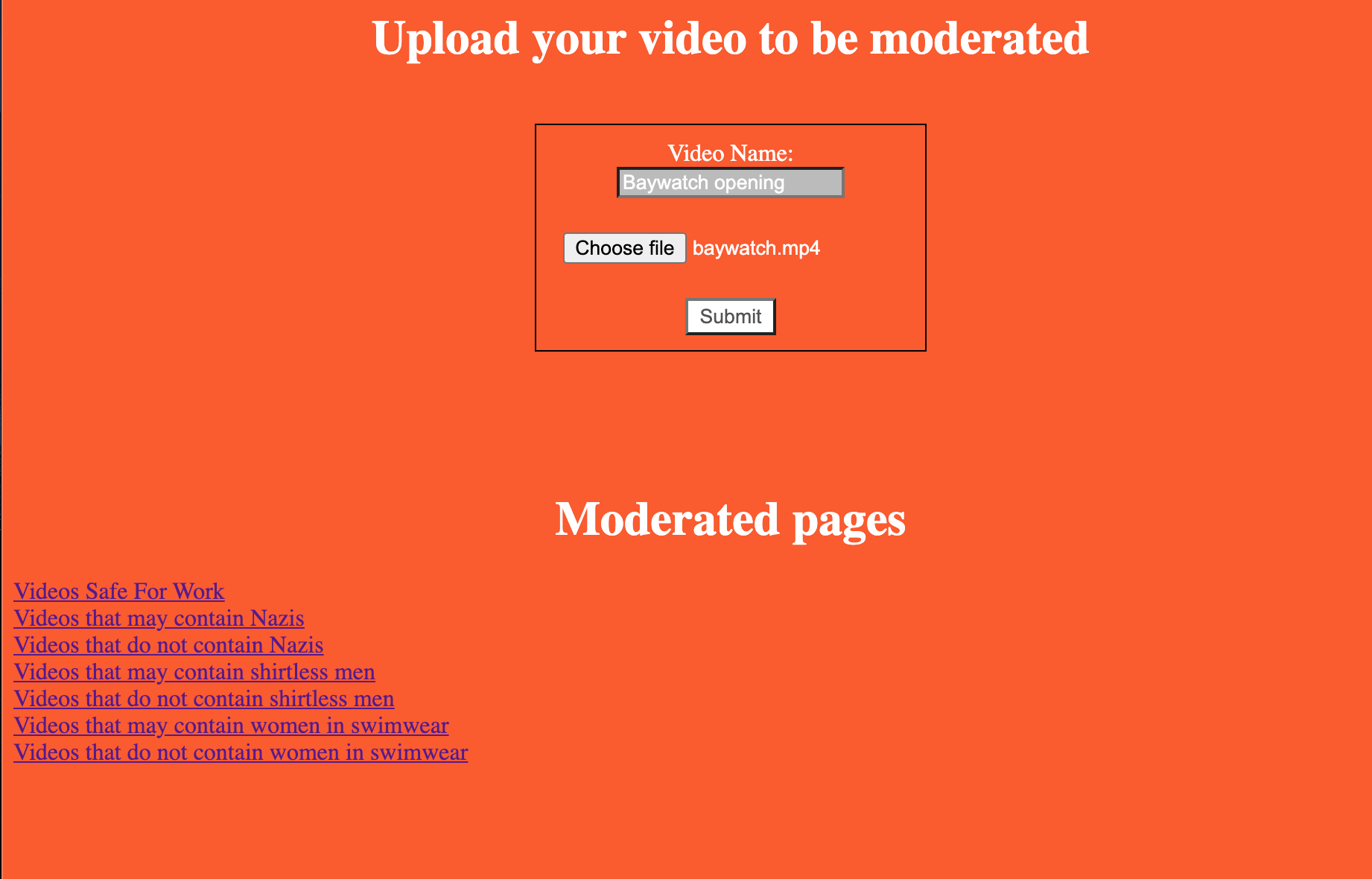
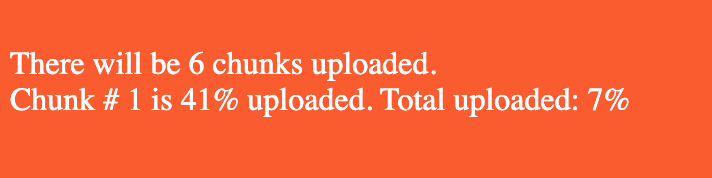
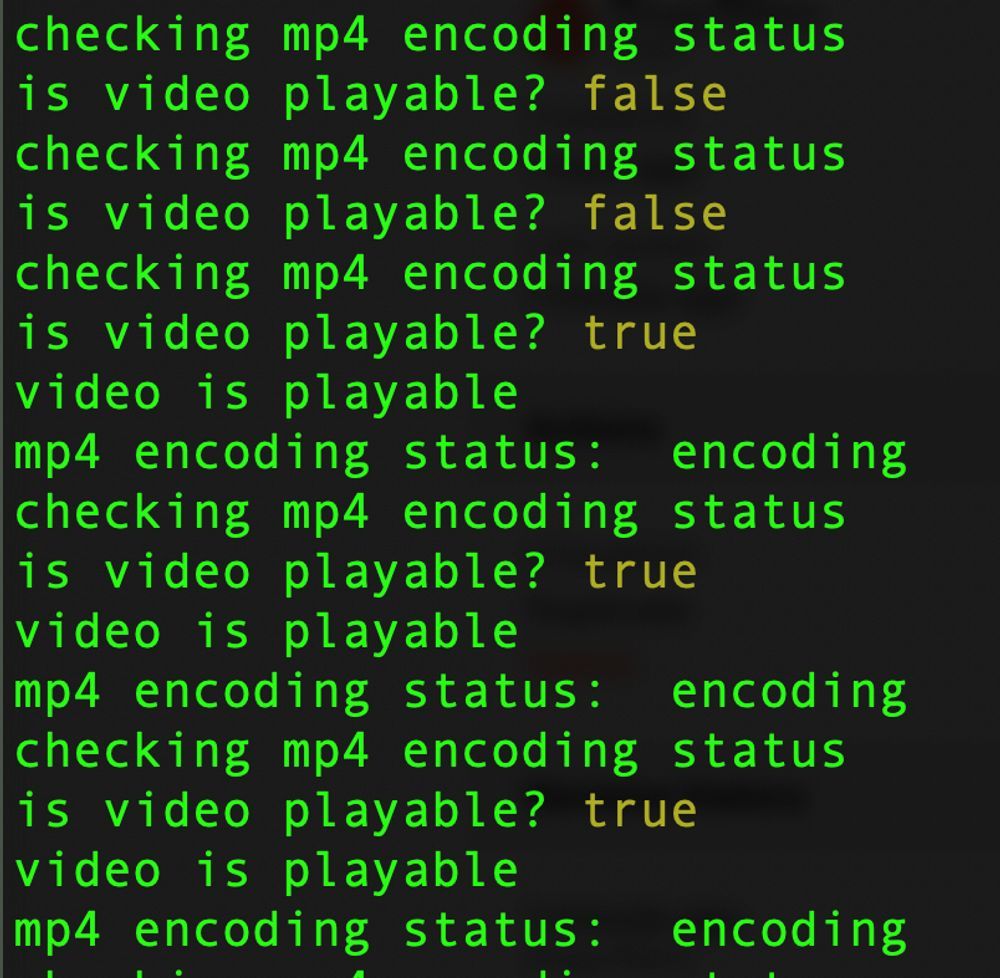
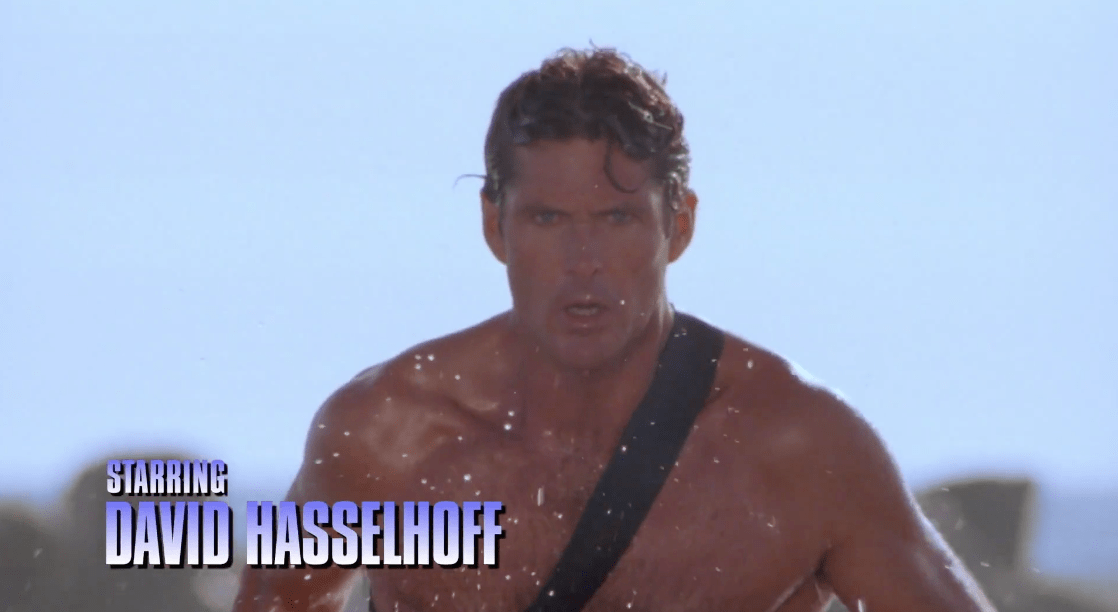
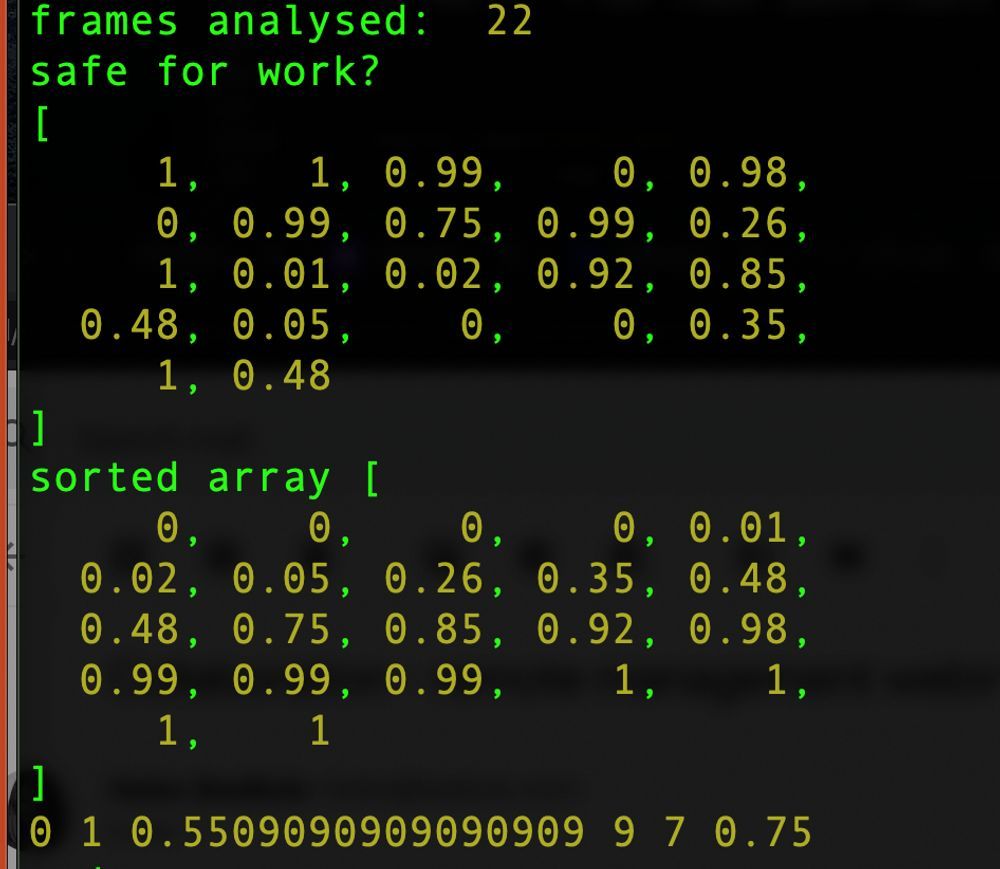
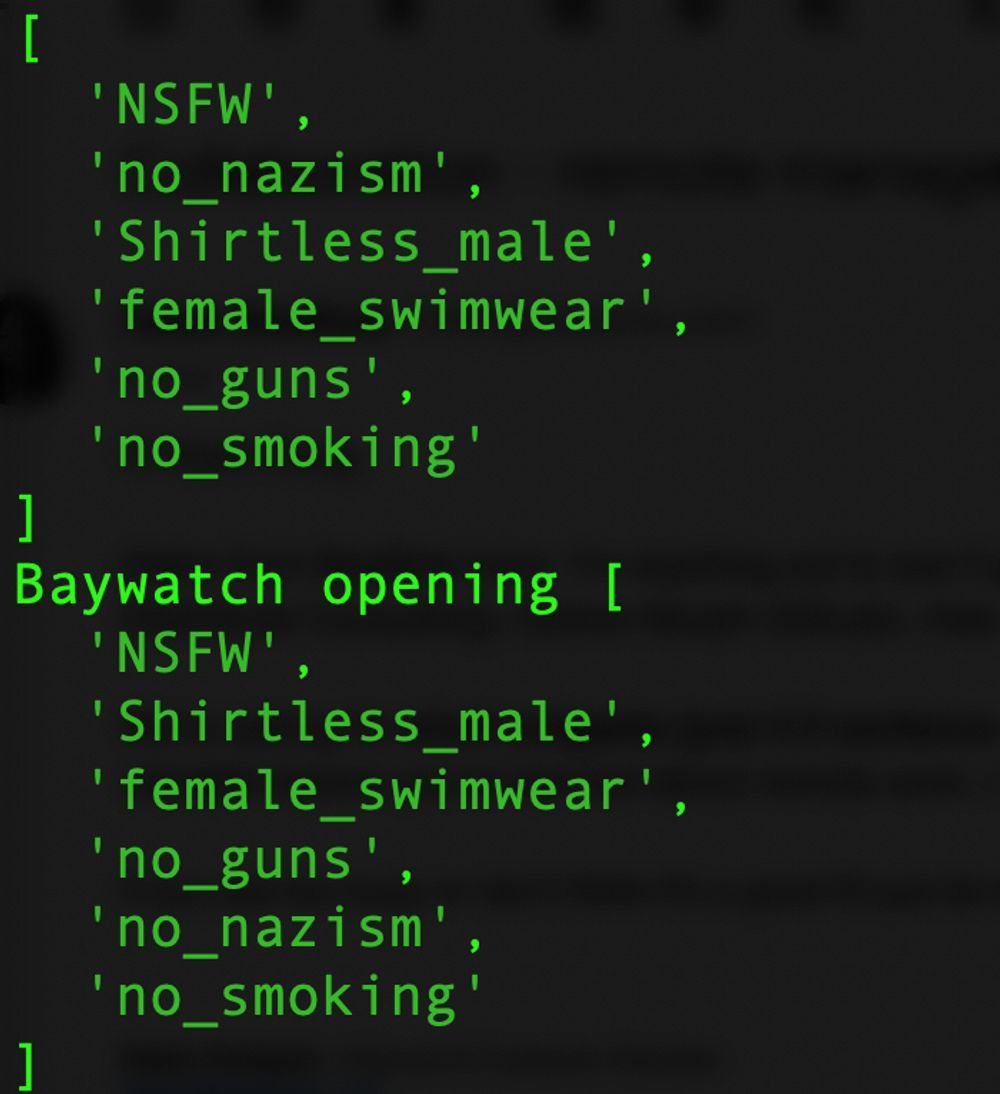
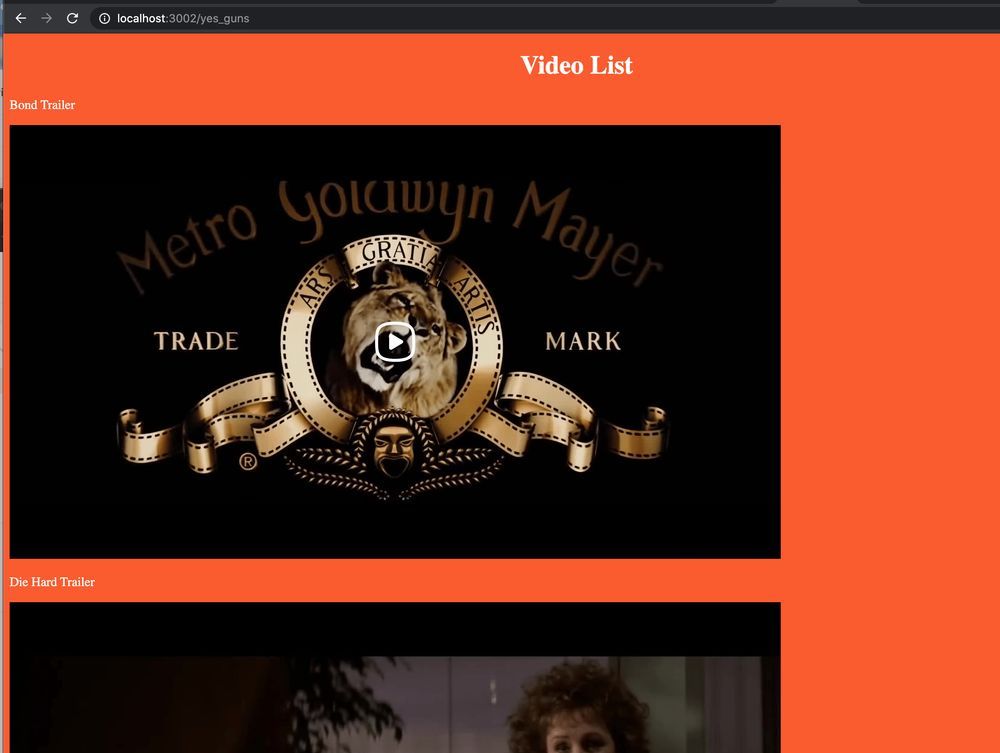
LATEST ARTICLES
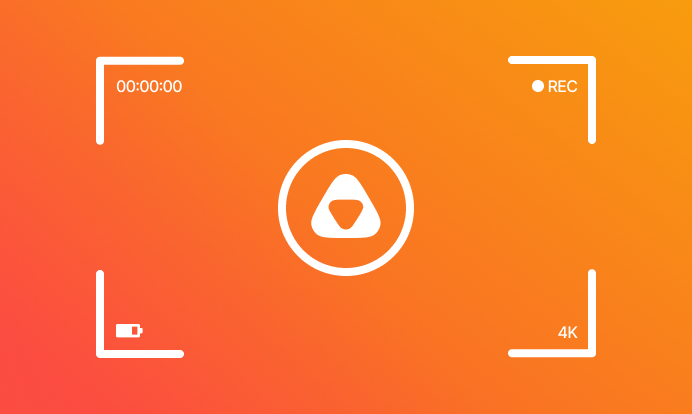
Video trends · 10 min read
Behind the scenes of video production at api.video — A tête-a-tête with our video editor
Go behind the scenes with our video editor at api.video! Discover the ins and outs of video production, from creative planning to final cut, and learn what it takes to bring great content to life.
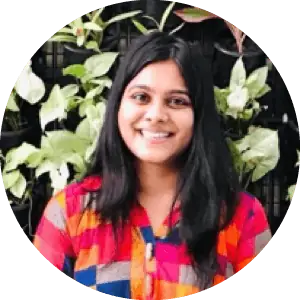
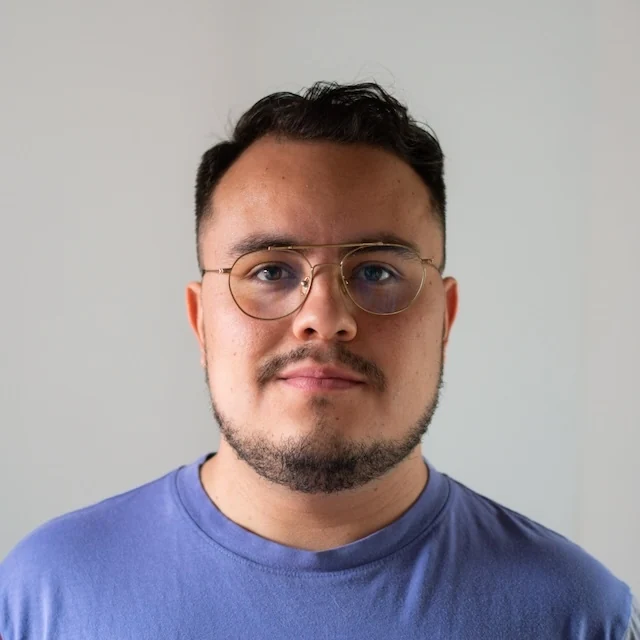
Multiple authors · November 12, 2024
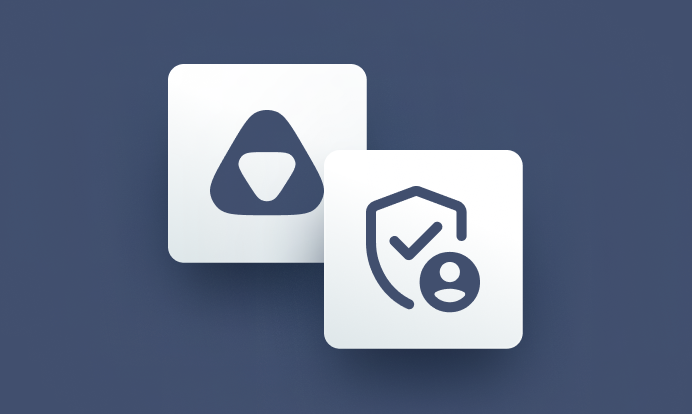
Video trends · 8 min read
Token-based authentication: The key to safer API interactions
Learn how token-based authentication in web APIs secures sensitive data and simplifies access control.
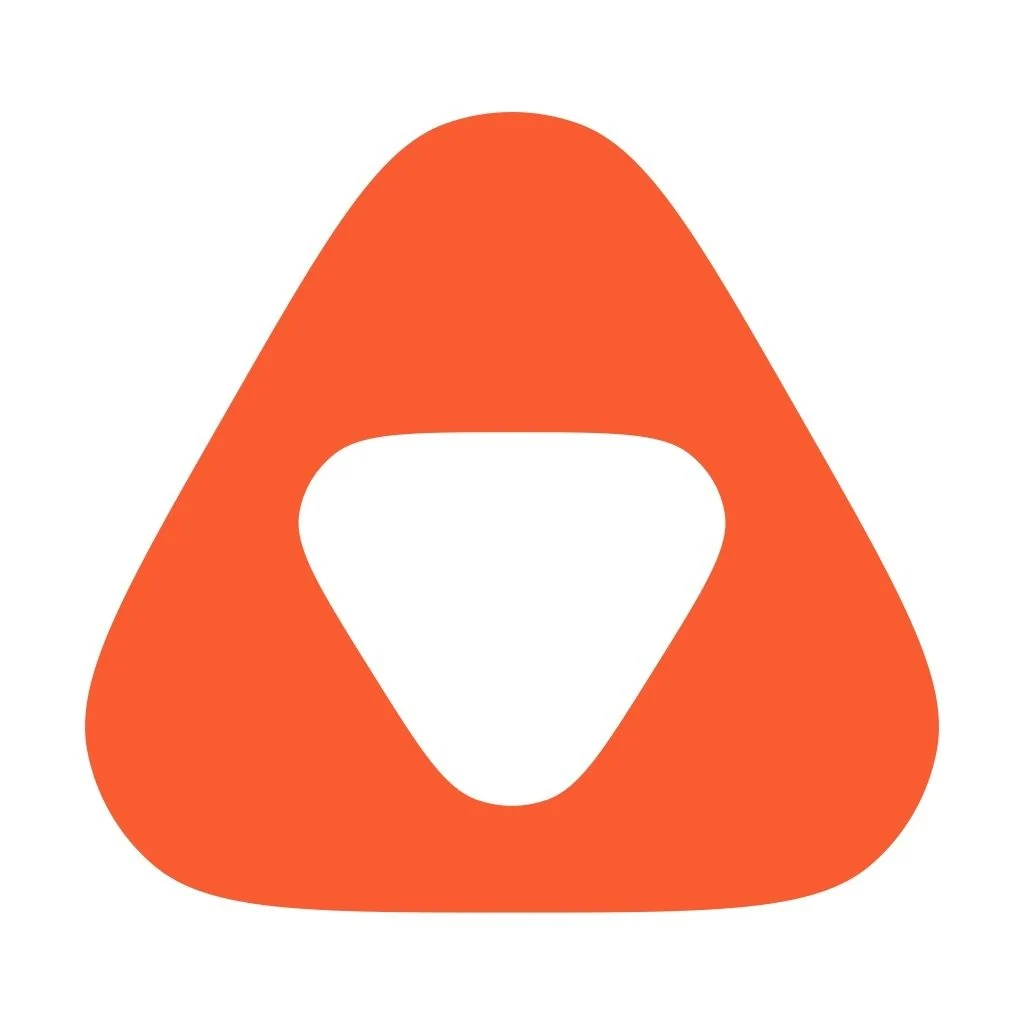
api.video · October 28, 2024
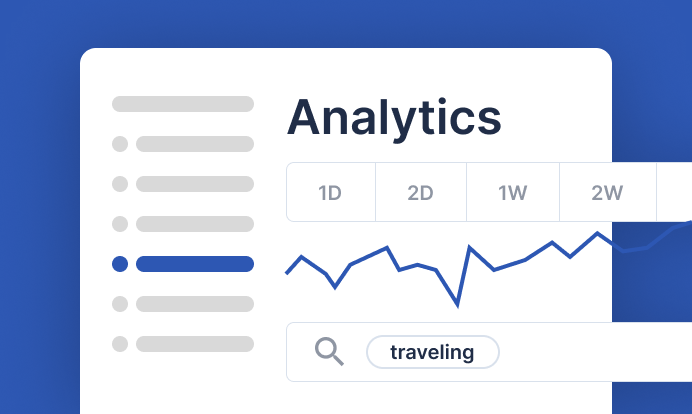
Video trends · 4 min read
How we use Analytics at api.video to optimize our video content — and you can too!
Learn practical tips and techniques to optimize your own video content with Analytics as we share the approach we use in-house.
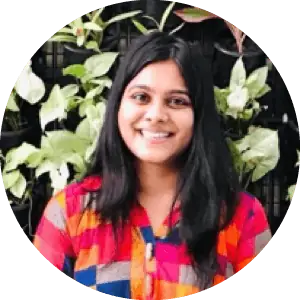
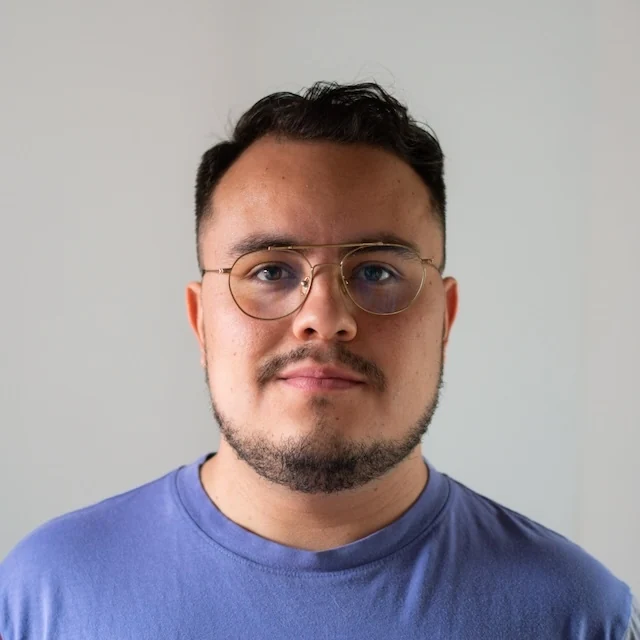
Multiple authors · October 18, 2024
Try out more than 80 features for free
Access all the features for as long as you need.
No commitment or credit card required
Video API, simplified
Fully customizable API to manage everything video. From encoding to delivery, in minutes.
Video API, simplified
Fully customizable API to manage everything video. From encoding to delivery, in minutes.
Built for Speed
The fastest video encoding platform. Serve your users globally with 140+ points of presence.
Built for Speed
The fastest video encoding platform. Serve your users globally with 140+ points of presence.
Let end-users upload videos
Finally, an API that allows your end-users to upload videos and start live streams in a few clicks.
Let end-users upload videos
Finally, an API that allows your end-users to upload videos and start live streams in a few clicks.
Affordable
Volume discounts and usage-based pricing to ensure you don’t exceed your budget.
Affordable
Volume discounts and usage-based pricing to ensure you don’t exceed your budget.